Common Functions
One of the most widely used functions is Comment().
Comment() Function
void Comment(...)
This function introduces a comment defined by a user into the upper left corner of a chart window. Parameters can be of any type. Number of parameters cannot exceed 64. Arrays cannot be passed to the Comment() function. Arrays should be introduced elementwise. Data of double type are written with 4 digits after the decimal point. For showing figures with accuracy use the DoubleToStr() function. Bool, datetime and color types will be written as digits. To show data of datetime type as a string use the TimeToStr() function.
Parameters:
... - any values separated by commas.
Example of using this function can be a simple EA
comment.mq4 reflecting the information about the number of orders.
int start()
{
int Orders=OrdersTotal();
if (Orders==0)
Comment("No orders");
else
Comment("Available ",Orders," orders." );
return;
}
At the program beginning the total number of orders is counted by the OrdersTotal() function. If the Orders variable (number of orders) is equal to 0, the Comment() function with "No orders" parameter is executed. If there is at least one order, Comment() with a list of parameters comma separated will be executed. In this case 3 parameters are used: the first one is a string value "Available", second is an integer value Orders and the third one is a string value "orders.". As a result of the function execution at each start of the special function start() one of the messages will be shown in the upper left corner of a chart window. Fig. 132 illustrates a chart window in the situation when there is one order present.
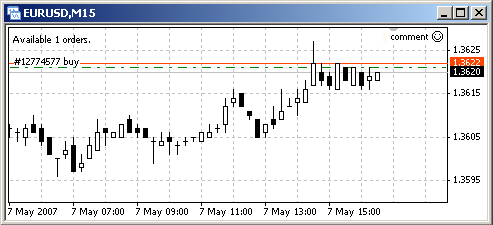
Fig. 132. Displaying a text in the upper left corner of a chart window as a result of Comment() execution.
For the reproduction of sound files PlaySound() function is used.
PlaySound() Function
void PlaySound(string filename)
The function plays a sound file. The file must be located in terminal_directory\sounds or its subdirectory.
Parameters:
filename - path to a sound file.
A set of recommended sound files can be found in the attachment - Sound Files.
In some cases a program can be written to support a dialog with a user. Function MessageBox() is used for this purpose.
MessageBox() function
int MessageBox(string text=NULL, string caption=NULL, int flags=EMPTY)
MessageBox function creates and displays a message box, it is also used to manage the dialog window. A message box contains a message and header defined ina program, as well as any combination of predefined icons and push buttons. If a function is executed successfully, the returned value is one of the return code values of MessageBox(). The function cannot be called from a custom indicator, because indicators are executed in the interface thread and may not decelerate it.
Parameters:
text - a text containing a message to be displayed;
caption - an optional text to be displayed in the message box. If the parameter is empty, an EA name will be displayed in the box header;
flags - optional flags defining the type and behavior of the dialog box. Flags can be a combination of flags from flag groups (see MessageBox Return Codes).
Let us consider an example of MessageBox() usage.
 |
Problem 31. Write a code of an EA displaying a message box with a question of closing all orders 5 minutes prior to important news release. If a user clicks Yes, all orders should be closed, if No is pushed, no actions should be performed.
|
The EA supporting a dialog with a user (dialogue.mq4) can have the following code:
#include <WinUser32.mqh>
extern double Time_News=15.30;
bool Question=false;
int start()
{
PlaySound("tick.wav");
double Time_cur=Hour()+ Minute()/100.0;
if (OrdersTotal()>0 && Question==false && Time_cur<=Time_News-0.05)
{
PlaySound("news.wav");
Question=true;
int ret=MessageBox("Time of important news release. Close all orders?",
"Question", MB_YESNO|MB_ICONQUESTION|MB_TOPMOST);
if(ret==IDYES)
Close_Orders();
}
return;
}
void Close_Orders()
{
Alert("Function of closing all orders is being executed.");
return;
}
In the block 1-2 WinUser32.mqh file is included into the program; in this file MessageBox() return codes are defined. Also in this block the external variable Time_news is introduced - this is the time of important news release. During the whole period of EA execution a question about closing orders should be displayed only once. To trace whether the question has been already displayed in the EA 'Question' variable is declared.
At each start of the special function start() (block 2-3) PlaySound() is executed. The played tick.wav sound resembles a weak click that denotes the best way the fact of a new tick appearance. The decision about using sound in a program is made by a programmer. In some cases it is very useful to use sounds. For example, a sound may denote the fact of an EA execution. Other sounds may correspond to other events, for example triggering of a trading criterion, order closing, etc.
Value of the actual variable Time_cur corresponds to the current server time. In the EA conditions, at which the message box must be displayed, are analyzed. If there is one or several orders, the message box has not been shown yet and the server time differs from important news release time by less than 5 minutes, certain actions are performed in the program. First of all function PlaySound() is executed, the played sound attracts the attention of a user. The Question flag gets the true value (do not show the next time). In the next line MessageBox() is executed:
int ret=MessageBox("Time of important news release. Close all orders?",
"Question", MB_YESNO|MB_ICONQUESTION|MB_TOPMOST);
In this case the value of a string constant "Time of important news release. Close all orders?" will be displayed in a dialog box, and "Question" value will be reflected in the upper box line. The MB_YESNO flag denotes the presence of buttons - in this case Yes and No buttons (see MessageBox Return Values). The MB_ICONQUESTION flag defines an icon displayed in the left part of the message box (each operating environment has its own set of icons, Fig. 133 illustrates an icon from Windows XP set). The MB_TOPMOST flag provides the box with the property "always on top", i.e. the box will be always visible irrespective of what programs are being executed at the moment on the computer. As a result of the execution of MessageBox() with indicated parameters a message box is displayed:
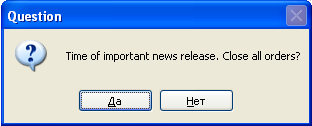
Fig. 133. Dialog box displayed as a result of MessageBox() execution.
At the moment when the message box is shown the program execution is held up until a user clicks on a button in the message box. As soon as it happens, control will be passed to the line following MessageBox() call, in this case to the block 3-4. This property of a message box to hold control is very important and it must be taken into account at a program development. For example, if a user left his computer and a message box was shown at this moment, during the whole time when a user is absent (until a button is pressed), the program will be waiting for response and no code will be executed in this period.
Note, before a message box is displayed the program execution is accompanied by a sound of ticks. When the message box is displayed another sound is played. In the period when the dialog box is open and waiting for response no sound is played which illustrates the fact of control holding while the dialog box is open. After a button is pressed, the program execution will continue and the sound of ticks will be played again.
If a user clicks Yes, the Close_Orders() function will be called; this function is used for closing orders. In this example the function contents is not described; to denote its execution the Alert function is executed ("Function of closing all orders is being executed."). If a user clicks No, the function of closing orders is not called. In the current session of the EA execution the message box will not be shown again.
Common Functions
Function
|
Summary Info |
Alert |
Displays a message box containing user-defined data. Parameters may be of any type. Number of parameters cannot exceed 64. |
Comment |
Displays a comment defined by a user in the upper loft corner of a chart window. Parameters may be of any type. Number of parameters cannot exceed 64. |
GetTickCount |
GetTickCount() returns number of milliseconds passed since a system was started. The counter is limited by the resolution of the system timer. Since time is stored as an unsigned integer, it is overfilled every 49.7 days. |
MarketInfo |
Returns various information about securities listed in "Market watch" window. Part of the information about the current security is stored in predefined variables (see MarketInfo() Identifiers). |
MessageBox |
MessageBox function creates and displays a message box, it is also used
to manage the dialog window. A message box contains a message and
header defined ina program, as well as any combination of predefined
icons and push buttons. If a function is executed successfully, the
returned value is one of the return code values of MessageBox(). The
function cannot be called from a custom indicator, because indicators
are executed in the interface thread and may not decelerate it. |
PlaySound |
Plays a sound file. The file must be located in the terminal_dir\sounds directory or in its subdirectory. |
Print |
Prints a message to the experts log. Parameters can be of any type. Amount of passed parameters cannot exceed 64. |
SendFTP |
Sends a file to the address specified in setting window of "Publisher" tab. If the attempt fails, it retuns FALSE.
The function does not operate in the testing mode. This function cannot be called from custom indicators, either.
The file to be sent must be stored in the terminal_directory\experts\files folder or in its sub-folders.
It will not be sent if there is no FTP address and/or access password specified in settings. |
SendMail |
Sends an email to the address specified in settings window of "Email" tab.
The sending can be disabled in settings, or it can be omitted to specify the e-mail address. |
Sleep |
The Sleep() function suspends execution of the current expert within the specified interval. Sleep() cannot be called from custom indicators, because indicators are executed in the interface thread and may not decelerate it.
The checking of the expert stop flag status every 0.1 second is built into the function. |
For the detailed description of these and other functions please refer to Documentation at MQL4.community, MetaQuotes Ltd. website or to "Help" section in MetaEditor.