Examples of implementation
In the previous section, we analyzed an example of special functions execution in a simple EA simple.mq4. For better practice, let us analyze some more modifications of this program.

|
Example of a correct program structure. |
As a rule, function descriptions are indicated in the same sequence as they are called for execution by the client terminal. Namely, first goes the description of init(), then start(), and the last one is deinit(). However, special functions are called for execution by the client terminal in accordance with their own properties. That is why the location of a description in a program does not matter. Let us change the order of descriptions and see the result (EA possible.mq4).
int Count=0;
int start()
{
double Price = Bid;
Count++;
Alert("New tick ",Count," Price = ",Price);
return;
}
int init()
{
Alert ("Function init() triggered at start");
return;
}
int deinit()
{
Alert ("Function deinit() triggered at exit");
return;
}
Starting this EA, you will see that the execution sequence of special functions in a program does not depend on the order of descriptions in a program. You may change the positions of function descriptions in a source code and the result will be the same as in the execution of the EA simple.mq4.

|
Example of incorrect program structure. |
But, the program will behave in a different way if we change the head part position. In our example we will indicate start() earlier than the head part (EA incorrect.mq4).
int start()
{
double Price = Bid;
Count++;
Alert ("New tick ",Count," Price = ",Price);
return;
}
int Count=0;
int init()
{
Alert ("Function init() triggered at start");
return;
}
int deinit()
{
Alert ("Function deinit() triggered at exit");
return;
}
When trying to compile this EA, MetaEditor will show an error message.
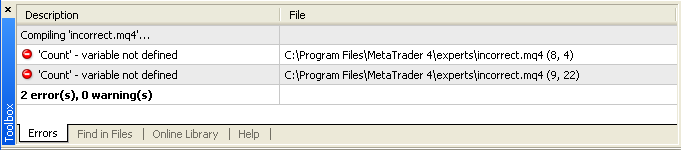
Figure 36 Error message during incorrect.mq4 program compilation.
In this case the line is written outside all functions, but is not at the very beginning of a program, but somewhere in the middle of it.
int Count=0;
The defining moment in the program structure is that the declaration of the global variable Count is done after function declarations (in our case, start()). In this section, we do not discuss the details of using global variables; types of variables and usage rules are described in Variables. It should be noted here that any global variable must be declared earlier (earlier in text) than the first call to it (in our case, it is in start()). In the analyzed program, this rule was violated, and the compiler displayed an error message.

|
Example of using a custom function. |
Now let us see how the program behaves in relation to custom functions. For this purpose, let us upgrade the code described in the example of a simple EA simple.mq4, and then analyze it. A program with a custom function will look like the following example (EA userfunction.mq4).
int Count=0;
int init()
{
Alert ("Function init() triggered at start");
return;
}
int start()
{
double Price = Bid;
My_Function();
Alert("New tick ",Count," Price = ",Price);
return;
}
int deinit()
{
Alert ("Function deinit() triggered at exit");
return;
}
int My_Function()
{
Count++;
}
First of all, let us see what has changed and what has remained unchanged.
Unchanged parts
1. The head part is unchanged.
int Count=0;
2. Special init() function is unchanged.
int init()
{
Alert ("Function init() triggered at start");
return;
}
3. Special deinit() function is unchanged.
int deinit()
{
Alert("Function deinit() triggered at exit");
return;
}
Changes
1. Custom function My_Function() is added.
int My_Function()
{
Count++;
}
2. The code of start() has also changed. Now, it contains the custom function call, and there is no Count variable calculation line.
int start()
{
double Price = Bid;
My_Function();
Alert("New tick ",Count," Price = ",Price);
return;
}
In Program execution we analyzed the order of init() and deinit() execution. In this example, these functions will be executed the same way, so we will not dwell on their operation. Let us analyze the execution of start() and My_Function(). The custom function description is located outside all special functions, as it must be. The custom function call is indicated within start(), which is also correct.
After init() is executed, the program will be executed in the following manner.
3.1.The special start() function is waiting to be started by the client terminal. When a new tick comes, the terminal will start this function for execution. As a result, the following actions will be performed.
3.2 (1).
double Price = Bid;
The same actions are performed in this line.
3.2.1(1). Local variable Price is initialized (see Types of variables). The value of this local variable will be available from any part of start().
3.2.2(1). The assignment operator is executed. The last available Bid will be assigned to Price (for example, at the first tick, it is equal to 1.2744).
3.3(1). Next comes the My_Function() call.
My_Function();
This line will be executed within the start() operation. The result of this function call is passing control to the function body (description), and returning it to the call place afterward.
3.4(1). There is only one operator in the custom function description.
Count++;
At the first custom function call, Count is equal to zero. As the result of the Count++ operator, Count increases by one. Having executed this operator (the only and the last one), the custom function finishes its operation and returns control to the place from which it has been called.
It should be noted here that custom functions may be called only from special functions, or from other custom functions that are called from special functions. That is why, at any current moment, one of the special functions is operating (or, start() is waiting for a new tick), and custom functions are executed only inside special functions.
In this case, control is returned to start(), which is being executed. That is, control is returned to the line following the function call.
3.5(1). This line contains an Alert() call.
Alert ("New tick ",Count," Price = ",Price);
Alert() will show in a window all constants and variables enumerated in brackets.
New tick 1 Price = 1.2744
3.6(1). The 'return' operator finishes start() operation.
return;
3.7. Control is passed to the client terminal waiting for a new tick.
At further start() executions, variables will get new values, and messages by Alert() will be shown. That is, the program will perform points 3.2 through 3.6. At each start() execution (at each tick), a call to My_Function() will be performed, and this function will be executed. The execution of start() will continue until a user decides to terminate the program operation. In this case, the special deinit() function will be executed, and the program will stop operating.
The program userfunction.ех4 started for execution will show a window containing messages by Alert(). Note, the result of the program operation will be the same as the result of a simple EA simple.mq4 operation. It is clear that the structure of userfunction.mq4 is made up in accordance with the usual order of functional blocks. If another acceptable order is used, the result will be the same.