Program structure
In the first sections, we cover some basic notions of the programming language MQL4. Now let us study how a program is organized in general. To solve this problem, we will study its structural scheme.
As mentioned above, the main program code written by a programmer is placed inside user-defined and special functions. In Functions, we discuss the notion and properties of built-in and user-defined functions. Briefly, a user-defined function has a description, and a function call is used for starting its execution in a program. A built-in or user-defined function can be executed only after it is called. In such a case, the function is said to be called for execution by a program.
Properties of special functions are described in detail in Special functions. Here, we study only the main information about them. A special function is a function called to be executed by the client terminal. Distinct from common functions, special functions only have a description, and special functions are not called from within a program. Special functions are called to be executed by the client terminal. (There is a technical possibility to call special functions from within a program, but we will consider this method incorrect and will not discuss it here.) When a program is started for execution in a security window, the client terminal passes control to one of the special functions. As a result, this function is executed.
The rule of programming in MQL4 is the following.

|
A program code must be written inside functions. |
It means program lines (operators and function calls) that are outside the special functions cannot be executed. At the attempt to compile such a program, MetaEditor will show the corresponding error message and the executable file *.exe will not appear as a result of compilation.
Let us consider the functional scheme of a common program, an EA.
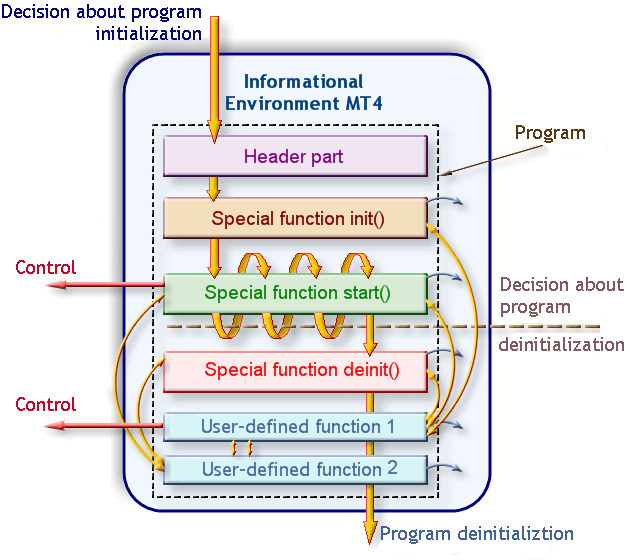
Figure 31 Functional scheme of a program (EA).
The largest blocks of a program written in MQL4 are:
- Head part of a program.
- Special init() function.
- Special start() function.
- Special deinit() function.
- User-defined functions.
Further, we will analyze only the inner content of these functional blocks (integral parts) of a program, while all external objects (for example, the informational sphere of the client terminal, or the hardware) will be out of our scope of interest.
Information environment of MetaTrader 4 Client Terminal
The information environment of MT4 is not a component part of the program. The information environment is a set of parameters available to be processed by a program. For example, it is the security price that has come with a new tick, the volume accumulated at each new tick, the information about maximum and minimum prices of history bars, the parameters that characterize trading conditions offered by a dealing center and so on. The information environment is always saved, and at each new tick, it is updated by the client terminal connected with the server.
Program structure
Head part
The head part consists of first lines at the beginning of a program, which contain general information about the program. For example, this part includes lines of declaration and initialization of global variables. (The necessity to include this or that information into the head part will be discussed later.) The sign of the head part end may be the next line containing a function description (user-defined or special function).
Special functions
Usually, after the head part, special functions are described. The special function description looks like the description of a user-defined function, but special functions have predefined names: init(), start() and deinit(). Special functions are a block of calculations and are related to the information environment of the client terminal and user-defined functions. Special functions are described in detail in Special functions.
User-defined functions
The descriptions of user-defined functions are usually given after the description of special functions. The number of user-defined functions in a program is unlimited. In Figure 31 previous, our example contains only two user-defined functions, but a program can contain 10 or 500, or none. If no user-defined functions are used in a program, the program will have a simple structure: the head part, and the description of special functions.
Standard functions
As mentioned previously, standard functions can be presented only as a function call. Each standard function, like special and custom functions, has a description, but this description is not given in a program (that is why not included in the scheme). The description of a standard function is hidden from the programmer, and it therefore cannot be changed. However, it is available to MetaEditor. During program compilation, MetaEditor will form an executable file, in which all called standard functions will be executed correctly to the full extent.
Arrangement of parts in a program
The head part should be located in the first lines. The arrangement of special and user-defined functions descriptions does not matter. Figure 32 shows a common arrangement of functional blocks, namely the head part, special functions, and user-defined functions. Figure 33 shows other program structure variants. In all examples, the head part comes first, while functions can be described in a random order.
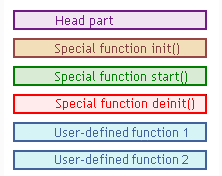
Figure 32 Usual arrangement of functional blocks in a program (recommended).
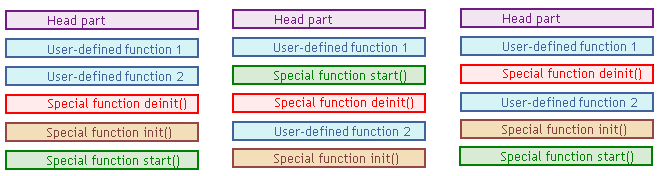
Figure 33 Possible ways of arranging functional blocks in a program (random order).

|
None of the functions can be described inside another function. It is prohibited to use in a program function descriptions located inside another function. |
Following are examples of incorrect arrangements of function descriptions.
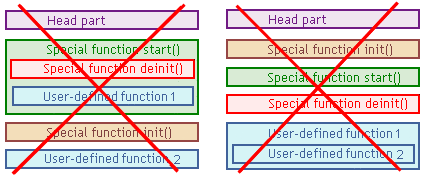
Figure 34 Examples of incorrect arrangement of function in a program.
If a programmer by mistake creates a program where the description of any of its functions is located inside the description of another function, then on the compilation stage, MetaEditor will show an error message and an executable file will not be created for such a program.
Code execution sequence
Head part and special functions
The moment a program is started in a security window, lines of the program head part are executed.
After the preparations described in the head part are done, the client terminal passes controlling to the special init() function, and that function is executed (control passing is shown at the structural scheme in large yellow arrows). The special init() function is called for execution only once at the beginning of the program operation. This function usually contains a code that should be executed only once before the main operation of the program starts. For example, when init() is executed, some global variables are initialized, graphical objects are displayed in a chart window, or messages may be shown. After all program lines in init() are executed, the function finishes its execution, and control is returned to the client terminal.
The main program operation time is the period of operation of the special start() function. In some conditions (see features of special functions in Special functions), including the receipt of a new tick by the client terminal from a server, the client terminal calls for execution of start(). This function (like other functions) can refer to the information environment of the client terminal, conduct necessary calculations, open and close orders, and soon. That is, it can perform any actions allowed by MQL4. When start() is executed, usually a solution is produced that is implemented as a control action (see the red arrows in Figure 31 previous). This control can be implemented as a trading request to open, close, or modify an order formed by the program.
After the whole code of the EA's special start() function is executed, start() finishes its operation and returns control to the client terminal. The terminal holds the control for some time, not starting any special functions. A pause appears, during which the program does not work. Later, when a new tick comes, the client terminal passes control to start() once again, and as a result, the function is executed again.
The process is repeated while the program is attached to a chart and can continue for weeks and months. During this period, an EA can conduct automated trading, that is it can implement its main assignment. In Figure 31 previous, the repeated call of start() is shown by several yellow arrows enveloping the special start() function.
When a trader removes an EA from a chart, the client terminal executes the special deinit() function once. The execution of this function is necessary for the correct termination of an EA's operation. During operation, a program may, for example, create graphical objects and global variables of the client terminal. The deinit() function can contain code to delete unnecessary objects and variables. After the execution of deinit() is completed, control is returned to the client terminal.
Executed special functions can refer to the information environment (see the thin blue arrows in Figure 31 previous) and call for execution of user-defined functions (see the thin yellow arrows in Figure 31 previous). Note that special functions are executed after they are called by the client terminal in the predefined order: first init(), then any number of calls of start() and after that deinit(). The conditions under which the client terminal calls special functions are described in Special functions.
User-defined functions
User-defined functions are executed when a call to that function is contained within some function. In this case, control is timely passed to the user-defined function, and after the function execution is over, control is returned to the place of the call (see the thin orange arrows in Figure 31 previous). The call of user-defined functions can be contained not only in the description of a special function, but also in the description of other user-defined functions. One user-defined function may call other user-defined functions. This is widely used in programming.
User-defined functions are not called for execution by the client terminal. Any user-defined functions are executed inside the execution of a special function that returns control to the client terminal. User-defined functions may also request for use the variable values of the client terminal information environment (see the thin blue arrows in Figure 31 previous).
If a program contains a description of a user-defined function, but there is no call of this function, this user-defined function will be excluded from the ready program on the compilation stage and will not be used in the program's operation.

|
Note: special functions are called for execution by the client terminal. User-defined functions are executed if called from special or other user-defined functions, but are never called by the client terminal. Control action (such as trading orders) can be executed both in special and user-defined functions. |