Program execution
Programming skills are better developed if a programmer has a small operating program at his disposal. To understand the whole program, it is necessary to examine thoroughly all its components and trace its operation step-by-step. Please note, special function properties of different application programs (EAs, scripts, indicators) are different. Here we will analyze how an EA operates.

|
Example of a simple EA. (simple.mq4) |
int Count=0;
int init()
{
Alert ("Function init() triggered at start");
return;
}
int start()
{
double Price = Bid;
Count++;
Alert("New tick ",Count," Price = ",Price);
return;
}
int deinit()
{
Alert ("Function deinit() triggered at deinitialization");
return;
}
In accordance with program execution rules (see Program structure and Special functions), this EA will work the following way:
1. At the moment when a program is attached to a chart, the client terminal passes control to the program, and as a result, the program starts its execution. The program execution starts from the head part. The head part contains only one line.
int Count=0;
In this line,the global variable Count is declared and initialized with a value of zero. (Local and global variables are analyzed in detail in Types of variables. It should be noted here that the algorithm used in the previous example program requires declaration of the variable Count as global. That is why it cannot be declared inside a function, it must be declared outside the function's description, that is in the head part; as a result, the value of the global variable Count will be available from any program part.)
2. After the execution of the program head part, init() will be started for execution. Please note, this function call is not contained in a program code. The start of init() execution when an EA is attached to a chart is the function's own property. The client terminal will call init() for execution just because the program code contains its description. In the analyzed program, the description of init() is the following.
int init()
{
Alert ("Function init() triggered at start");
return;
}
The function body contains only two operators.
2.1 Function Alert() shows an alert window:
Function init() triggered at start
2.2 Operator return finishes the operation of init().
As a result of init() execution, an alert will be written. In commonly used programs, such an algorithm is very rare, because such init() usage is of little help. Really, there is no sense to use a function that informs a trader that it is being executed. Here, the algorithm is used only for the visualization of init() execution. Pay attention to the fact that init() is executed in a program only once. The function execution takes place at the beginning of program operation after a head part has been processed. When the operator return is executed in the special init() function, the program returns control to the client terminal.
3. The client terminal detects the description of start() in the program.
int start()
{
double Price = Bid;
Count++;
Alert("New tick ",Count," Price = ",Price);
return;
}
3.1. Control is held by the client terminal. The client terminal waits for a new tick and does not start program functions until a new tick comes. It means that the program does not operate for some time, that is no actions are performed in it. A pause appears, though there is neither direct nor indirect prompting to perform this pause. The necessity to wait for a tick is the start() function's own property, and there is no way of a program influence upon this property (for example, disabling it). The program will be waiting for the control until a new tick comes. When a new tick comes, the client terminal passes control to the program, namely to start() (in this case in accordance with the EA's start() function property). As a result, its execution starts.
3.2.1.
double Price = Bid;
The following actions are performed.
3.2.1.1. Declaration of a local variable Price (see Types of variables). The value of this local variable will be available to anything within start().
3.2.2. Execution of the assignment operator. The current Bid value will be assigned to Price. A new price value appears each time a new tick comes. (For example, at the first tick a security price can be equal to 1.2744.)
3.3. Then, the following line is executed.
Count++;
Count=Count+1; is another way to write this.
At the moment of passing control to this line, Count is equal to zero. As a result of Count++ execution, the value of Count will increase by one. So, at the moment of passing control to the next line, Count will be equal to 1.
3.4. The next line contains an Alert() function call.
Alert("New tick ",Count," Price = ",Price);
The function will write all constants and variables enumerated in brackets.
At the first execution of start(), the program will write "New tick", then refer to Count to get its value (at first execution, this value is one), write this value, then write " Price = " and refer to Price to get its value (in our example it is 1.2744), and finally write that value.
As a result, the following line will be written:
New tick 1 Price = 1.2744
3.5. The 'return' operator finishes the operation of start().
return;
3.6. Control is returned to the client terminal until a new tick comes.
This is how the start() function of an EA is executed. When the execution is over, start() returns control to the client terminal, and when a new tick comes, the client terminal calls start() again. This process (starting the execution of the start() function and returning control to the client terminal) can go on for a long timeseveral days or weeks. During all this time the special start() function will be executed from time to time. Depending on environment parameters (new prices, time, trade conditions and so on), start() can perform various actions like opening or modifying orders.
3.7. From the moment of receipt of a new tick, actions of points 3.2 through 3.6 are repeated. However, only the sequence of executed operators is repeated, but variables get new values each time. Let us view the differences between the first and the second execution of start().
3.2.1(2).
double Price = Bid;
The following actions are performed
3.2.1.1(2). Declaration of a local variable Price (unchanged).
3.2.2(2). Execution of the assignment operator. The current Bid value will be assigned to Price. A new price value appears each time a new tick comes. For example, at the second tick, the security price is equal to 1.2745.
3.3(2). The following line will be executed.
Count++;
The moment prior to passing control to this line, the value of Count (after the first execution of the start() function) is equal to one. As a result of Count++ execution, Count increases by one. Thus, at the second execution, Count will be equal to to (changed).
3.4(2). Alert() writes all constants and variables (their new values) enumerated in brackets.
Alert("New tick ",Count," Price = ",Price);
At the second start() execution, the program will write "New tick ", then refer to Count to get its value (in the second execution, it is equal to two), write this value, write " Price = ", refer to Price to get its value (in our example 1.2745), and write that value (changed).
As a result, the following line will be written:
New tick 2 Price = 1.2745
3.5(2). The 'return' operator finishes the operation of start() (no changes).
return;
3.6(2). Control is returned to the client terminal to wait for a new tick.
3.7(2). Then it is repeated again. In the third start() execution, variables will get new values and will be written by Alert(). That is, the program repeats points 3.2 through 3.6(3). And, then again and again: 3.2-3.6(4), 3.2-3.6(5),..(6)..(7)..(8)... If a user does not take any actions, this process will be repeated endlessly. As a result of the start() operation in this program, we will see the tick history of price changes.
The next events will happen only when a user decides to terminate the program and forcibly detaches the program from a chart manually.
4. The client terminal passes control to deinit() (in accordance with its properties).
int deinit()
{
Alert ("Function deinit() triggered at exit");
return;
}
There are only two operators in the function body.
4.1. Alert() will write:
Function deinit() triggered at deinitialization
4.2. The 'return' operator finishes the operation of deinit().
The deinit() function is started for execution by the client terminal only once. After that, the previous alert will appear, and the program will be removed from the chart.
5. Here the story of EA execution ends.
Attach this example program to any chart, and start it. The operating program will display a window containing all alerts generated by Alert(). By the contents of the alerts, it is easy to understand what special function is connected with this or that entry.
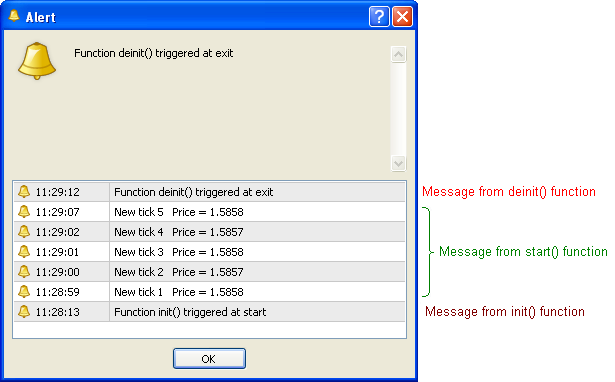
Figure 35 Results of operation of the program simple.mq4.
From this example, you can easily see that a program is executed in accordance with the special functions properties described in Special functions. Terminate the program, and start it again. Having done this several times, you will get experience using your first program. It will work both now and the next time. Further programs that you will write yourself will also be constructed in accordance with the described structure, and to start their execution you will also need to attach it to a chart.
If you understand all the concepts and rules, then the process of creating programs in MQL4 will be easy and pleasant.