Types of Variables
An application program in MQL4 can contain tens and hundreds of variables. A very important property of each variable is the possibility to use its value in a program. The limitation of this possibility is connected with the variable scope.
Variable scope is a location in a program where the value of the variable is available. Every variable has its scope. According to scope there are two types of variables in MQL4: local and global.
Local and Global Variables
Local variable is a variable declared within a function. The scope of local variables is the body of the function, in which the variable is declared. Local variable can be initialized by a constant or an expression corresponding to its type.
Global variable s a variable declared beyond all functions. The scope of global variables is the entire program. A global variable can be initialized only by a constant corresponding to its type (and not expression). Global variables are initialized only once before stating the execution of special functions.
If control in a program is inside a certain function, values of local variables declared in another function are not available. Value of any global variable is available from any special and user-defined functions. Let's view a simple example.
 |
Problem 22. Create a program that counts ticks. |
Solution algorithm of Problem 22 using a global variable (countticks.mq4):
int Tick;
int start()
{
Tick++;
Comment("Received: tick No ",Tick);
return;
}
In this program only one global variable is used - Tick. It is global because it is declared outside start() description. It means the variable will preserve its value from tick to tick. Let's see the details of the program execution.
In Special Functions we analyzed criteria at which special functions are started. Briefly: start() in Expert Advisors is started by a client terminal when a new tick comes. At the moment of the Expert Advisor's attachment to a security window, the following actions will be performed:
1. Declaration of the global variable Tick. This variable is not initialized by a constant, that is why its value at this stage is equal to zero.
2. Control is held by the client terminal until a new tick comes.
3. A tick is received. Control is passed to the special function start().
3.1. Inside start() execution control is passed to the operator:
Tick++;
As a result of the operator execution Tick value is increased by 1 (whole one).
3.2. Control is passed to the operator:
Comment("received: tick No ",Tick);
Execution of the standard function Comment() will cause the appearance of the alert:
3.3. Control is passed to the operator:
return;
As a result of its execution start() finishes its operation, control is returned to the client terminal. The global variable continues existing, its value being equal to 1.
Further actions will be repeated starting from point 2. Variable tick will be used in calculations again, but on the second tick at the starting moment of the special function start() its value is equal to 1, that is why the result of the operator
Tick++;
execution will be a new value of the variable Tick - it will be increased by 1 and now will be equal to 2 and execution of Comment() will show the alert:
Thus Tick value will be increased by 1 at each start of the special function start(), i.e. at each tick. The solution of such problems is possible only when using variables that preserve their values after exiting a function (in this case a global variable is used). It is unreasonable to use local variables for this purpose: a local variable loses its value a function, in which it is declared, finishes its operation.
It can be easily seen, if we start an Expert Advisor, in which Tick is opened as a local variable (i.e. the program contains an algorithmic error):
int start() // Special function start()
{
int Tick; // Local variable
Tick++; // Tick counter
Comment("Received: tick No ",Tick);// Alert that contains number
return; // start() exit operator
}
From the point of view of syntax there are no errors in the code. This program can be successfully compiled and started. It will operate, but each time the result will be the same:
It is natural, because variable Tick will be initialized by zero at the beginning of the special function start() at its each start. Its further increase by one will result in the following: by the moment of alert Tick value will always be equal to 1.
Static Variables
On the physical level local variables are presented in a temporary memory part of a corresponding function. There is a way to locate a variable declared inside a function in a permanent program memory - modifier 'static' should be indicated before a variable type during its declaration:
static int Number; // Static variable of integer type
Below is the solution of Problem 22 using a static variable
(Expert Advisor
staticvar.mq4 ):
int start()
{
static int Tick;
Tick++;
Comment("Received: tick No ",Tick);
return;
}
Static variables are initialized once. Each static variable can be initialized by a corresponding constant (as distinct from a simple local variable that can be initialized by any expression). If there is no explicit initialization, a static variable is initialized by zero. Static variables are stored in a permanent memory part, their values are not lost at exiting a function. However, static variables have limitations typical of local variables - the scope of the variable is the function, inside which the variable is declared, as distinct from global variables whose values are available from any program part. You see, programs
countticks.mq4 and staticvar.mq4 give the same result.
All arrays are initially static, i.e. they are of static type, even if it is not explicitly indicated (see Arrays).
External Variables
External variable is a variable, the value of which is available from a program properties window. An external variable is declared outside all functions and is a global one - its scope is the whole program. When declaring an external variable, modifier 'extern' should be indicated before its value type:
extern int Number; // External variable of integer type
External variables are specified in the program head part, namely before any function that contains an external function call. Use of external variables is very convenient if it is needed from time to time to start a program with other variables values.
 |
Problem 23. Create a program, in which the following conditions are implemented: if a price reached a certain Level and went down this level in n points, this fact should be once reported to a trader. |
Obviously, this Problem implies the necessity to change settings, because today's prices differ from ones that were yesterday; as well as tomorrow we will have different prices. To provide the option of changing settings in the Expert Advisor
externvar.mq4
external variables are used:
extern double Level = 1.2500;
extern int n = 5;
bool Fact_1 = false;
bool Fact_2 = false;
int start()
{
double Price = Bid;
if (Fact_2==true)
return;
if (NormalizeDouble(Price,Digits) >= NormalizeDouble(Level,Digits))
Fact_1 = true;
if (Fact_1 == true && NormalizeDouble(Price,Digits)<=
NormalizeDouble(Level-n*Point,Digits))
My_Alert();
return;
}
void My_Alert()
{
Alert("Conditions implemented");
Fact_2 = true;
return;
}
In this program external variables are set up in the lines:
extern double Level = 1.2500;
extern int n = 1;
Values of external variables are available from a program parameters window. The asset of these variables is that they can be changed at any moment - on the stage of attaching the program to a security window and during the program operation.
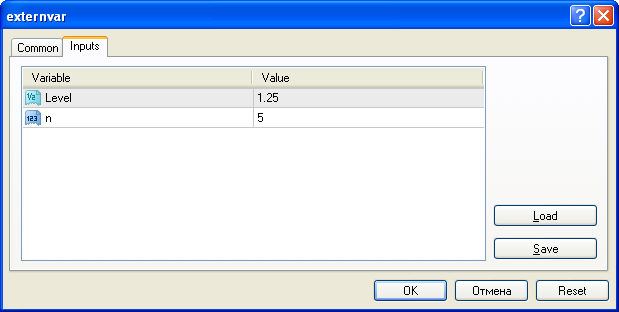
Fig. 54. Program properties window; here values of variables can be changed.
At the moment of attaching the program to a security window, variable values contained in the program code will be indicated in a program parameters window. A user can change these values. From the moment when a user clicks OK, the program will be started by the client terminal. The values of external variables will be those indicated by a user. In the operation process these values can be changed by the executed program.
If a user needs to change values of external variables during the program's operation, setup window should be opened and changes should be made. It must be remembered that program properties toolbar can be opened only in the period when the program (Expert Advisor or indicator) is waiting for a new tick, i.e. none of special functions is executed. During the program execution period this tollbar cannot be opened. That is why if a program is written so, that it is executed long time (several seconds or tens of seconds), a user may face difficulties trying to access the parameters window. Values of external variables of scripts are available only at the moment of attaching the program to a chart, but cannot be changed during operation If the parameters window is open, Expert Advisor does not work, control is performed by the client terminal and is not passed to a program for starting special functions.
 |
Please note, that when an EA properties window is open and a user is making decision about external variable values, the EA (or indicator) does not work. Having set the values of external variables and clicking OK the user starts the program once again. |
The client terminal starts successively the execution of the special function deinit(), then the special function init(), after that when a new tick comes - start(). At the execution of deinit() that finishes a program, external variables will have values resulted from the previous session, i.e. those available before the EA settings tollbar was opened. Before the execution of init() external variables will get values setup by a user in the settings toolbar and at the execution of init() external variables will have new values set by a user. Thus new values of external variables become valid from the moment of a new session (init - start - deinit) of an Expert Advisor that starts from the execution of init().
The fact of opening a setup window does not influence values of global variables. During all the time when the window is open and after it is closed, global variables preserve their values that were valid at the moment preceding the toolbar opening.
In the program externvar.mq4 also one local and two global variables are used.
bool Fact_1 = false;
bool Fact_2 = false;
double Price = Bid;
Algorithmically the problem solution looks like this. Two events are identified: the first one is the fact of reaching Level, the second - the fact that the alert (about getting lower than Level in n points) has been shown. These events are reflected in the values of variables Fact_1 and Fact_2: if the event did not happen, the value of the corresponding value is equal to false, otherwise - true. In the lines:
if (NormalizeDouble(Price,Digits) >= NormalizeDouble(Level,Digits))
Fact_1 = true;
the fact of the first events happening is defined. The standard function NormalizeDouble()
allows to conduct calculations with values of actual variables at a set accuracy (corresponding to the accuracy of a security price). If the price is equal to or higher than the indicated level, the fact of the first event is considered to be fulfilled and the global variable Fact_1 gets the true value. The program is constructed so that if Fact_1 once gets the true value, it will never be changed into false - there is no corresponding code in the program for it.
In the lines:
if (Fact_1 == true && NormalizeDouble(Price,Digits)<=
NormalizeDouble(Level-n*Point,Digits))
My_Alert();
the necessity to show an alert is defined. If the first fact is completed and the price dropped by n points (less or equal) from an indicated level, an alert must be shown - user-defined function My_Alert() is called. In the user-defined function after the alert the fact of already shown alert is indicated by assigning 'true' to the variable Fact_2. Then the user-defined function and after it the special function start() finish their operation.
After the variable Fact_2 gets the true value, the program will each time finish its operation, that's why a once shown alert will not be repeated during this program session:
if (Fact_2==true)
return;
Significant in this program is the fact that values of global variables can be changed in any place (both in the special and the user-defined functions) and are preserved within the whole period of program operation - in the period between ticks, after changing external variables or after changing a timeframe.
In general case values of global variables can be changed in any special function. That is why one should be extremely attentive when indicating operators that change values of global variables in init() and deinit(). For example, if we zero the value of a global variable in init(), at the first start() execution the value of this variable will be equal to zero, i.e. the value acquired during the previous start() execution will be lost (i.e. after changing external program settings).