Date and Time
The online trading system MetaTrader 4 uses the indications of two time sources - the local (PC) time and the server time.
Local time - the time that is set on the local PC.
Server time - the time that is set on the server.
TimeLocal() Function
datetime TimeLocal()
The function returns the local PC time expressed in the number of
seconds lapsed since 00:00 of the 1st of January 1970. Note: At
testing, the local time is modeled and coincides with the modeled
last-known server time.
A large majority of events that take place in the client terminal
are considered with accordance to the server time. The time of tick coming, new bar beginning, order opening and closing is considered
with accordance to the server time. To get the value of the server time
that corresponds with the current time, the TimeCurrent() function
should be used:
TimeCurrent() Function
datetime TimeCurrent()
The function returns the last known value of the server time (the
time of the last quote coming) expressed in seconds lapsed since 00:00
of the 1st of January 1970. The client terminal updates the time of the
last quote coming (together with other environment variables) before
launching special functions for execution. Each tick is characterized
with its own value of the server time that can be obtained using the
TimeCurrent() function. During the execution, this value can only be
changed as a result of the RefreshRates() function call and only if the
information has been updated since the last execution of the
RefreshRates() function, i.e., in case the new values of some
environment variables have come from the server.
The time of bar opening, Time[i], does not coincide with the time of
new tick coming, as a rule. The time of any timeframe bar opening is
always divisible by the timeframe. Any first tick appeared within a
timeframe is bar-forming; if there is no tick receipt within a
timeframe, the bar will not be formed within the timeframe.
For example, the tick coming to the terminal at time (server) t0
results in forming a bar with the time opening equal to Time[i+2] (Fig.
143). The moment specified as the beginning of the timeframe does not
concur with moment t0, though it can accidentally concur with it, in
general. The subsequent ticks that come to the terminal within the same
timeframe (at the moments of t1 and t2) can change the parameters of
the bar, for example, maximum price or open price, but they do not
affect the time of bar opening. The bar closing time is not considered
in the online trading system MetaTrader 4 (formally, the time of the
last tick coming within a timeframe or the beginning time of the next
timeframe can be considered as the bar closing time, as shown in Fig.
143).
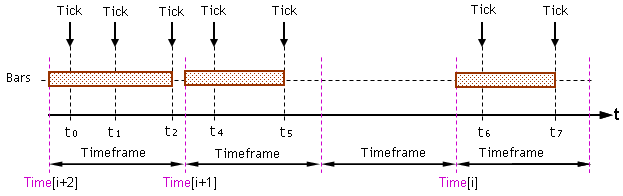
Fig. 143. Bar forming sequence in the online trading platform MetaTrader 4.
It is shown in Fig. 143 that it is possible that bars are not formed
at some time periods that are equal to the timeframe. Thus, between
time t5 of the tick coming and t6 of the next tick coming, the full
timeframe is packed, so the new bar hasn't been formed at that time
period. In this manner, the time of bar opening may differ from the
time of opening of an adjacent bar by more than a whole timeframe, but
it is always divisible by a timeframe. To demonstrate the sequence of
bar forming, we can use the EA timebars.mq4 that outputs the time of tick coming and the time of bar opening:
int start()
{
Alert("TimeCurrent=",TimeToStr(TimeCurrent(),TIME_SECONDS),
" Time[0]=",TimeToStr(Time[0],TIME_SECONDS));
return;
}
The results of the EA timebars.mq4
working are shown in Fig. 144. It is obvious that the first tick at the
regular time period of 1 minute duration came at 14:29:12, at the same
time a new bar was formed with the opening time - 14:29:00. Please note
that the right column of the message box displays the server time, the
left column displays the local time.
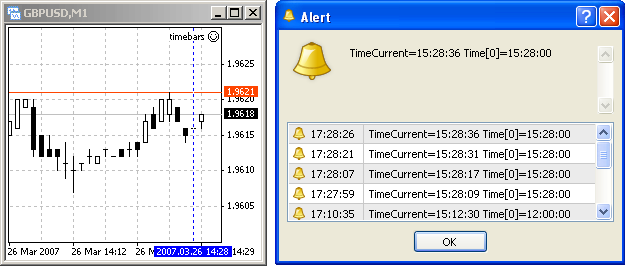
Fig. 144. Bar forming sequence in the online trading system MetaTrader 4.
In case the ticks come rarely (for example, the period between the
end of the European session and the beginning of the Asian session),
you can observe another phenomenon during the execution of timebars.mq4:
the opening time of the adjacent bars can differ from each other by
more than 1 minute (for one-minute timeframe). At the same time, the
indexing of bars is saved in succession, without spaces.
The server time of servers in different dealing centers may vary.
The time of beginning and finishing trades is set on each server
individually and it can disagree with the begining and the end of the
regular day. Some dealing centers, for example, have the settings that
perform trade opening on Sunday at
23:00 of server time. This results in forming of incomplete daily bars,
their practical duration is equal to one hour (Fig. 145).
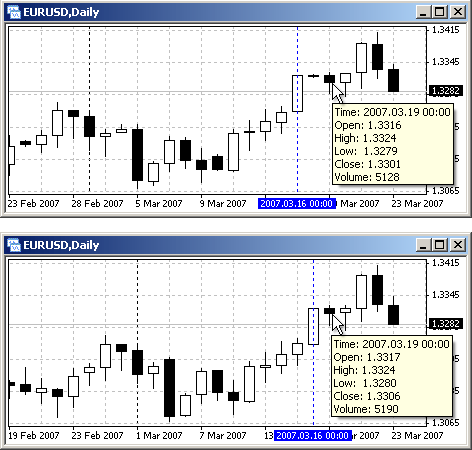
Fig. 145. Different bar history in different dealing centers.
The usage of date and time functions is rather easy in MQL4. Some of
them transform the server and the local time in seconds lapsed since
00:00 of the 1st of January 1970 into an integer number that
corresponds with an hour, a day, etc. Other functions return an integer
number that corresponds with the current hour, day, minute, etc.
TimeSeconds (), TimeMinute(), TimeHour(), TimeDay(), TimeMonth(), TimeYear(), TimeDayOfWeek () and TimeDayOfYear() Functions
This is a group of functions that return the number of seconds
lapsed from the beginning of the minute, or minute, hour, day, month,
year, day of week and day of year for the specified time. For example:
int TimeMinute(datetime time)
The function returns minutes for the specified time.
Parameters:
time - the date expressed in number of seconds that lapsed since 00:00 of the 1st of January 1970.
int TimeDayOfWeek(datetime time)
This function returns the day of week (0-Sunday,1,2,3,4,5,6) for the specified date.
Parameters:
time - the date expressed in number of seconds that lapsed since 00:00 of the 1st of January 1970.
The considered functions can be used for analysis of any bar opening time, for example. The EA named
bigbars.mq4 intended for finding bars of a size that is not less than specified size is shown below.
extern int Quant_Pt=20;
int start()
{
int H_L=0;
for(int i=0; H_L<Quant_Pt; i++)
{
H_L=MathAbs(High[i]-Low[i])/Point;
if (H_L>=Quant_Pt)
{
int YY=TimeYear( Time[i]);
int MN=TimeMonth( Time[i]);
int DD=TimeDay( Time[i]);
int HH=TimeHour( Time[i]);
int MM=TimeMinute(Time[i]);
Comment("The last price movement more than ",Quant_Pt,
" pt happened ", DD,".",MN,".",YY," ",HH,":",MM);
}
}
return;
}
The bigbars.mq4
EA searches the nearest bar whose height (difference between minimum
and maximum) is more than or equal to the value specified in the
external variable Quant_Pt. The date and time of the found bar are
outputted to the window of financial instrument by the Comment()
function.
Seconds (), Minute(), Hour(), Day(), TimeMonth(), TimeYear(), DayOfWeek () and DayOfYear() Functions
This is the group of functions that return the current second,
minute, hour, day, month, year, day of week and day of year for the
last known server time. The last known server time is the server time
that corresponds with the moment of the program launch (launch of any
special function by the client terminal). The server time is not
changed during the execution of the special function.
int Hour()
It returns the current hour (0,1,2,..23) of the last known server
time. Note that the last known server time is modeled during testing.
int DayOfYear()
It returns the current day of the year (1 is the 1st of
January,..,365(6) is the 31st of December), i.e., the day of the year
of the last known server time. Note that the last known server time is
modeled during testing.
The EA timeevents.mq4 that performs some actions as soon as the specified time comes can be used as an example of usage of the above functions.
extern double Time_Cls=16.10;
bool Flag_Time=false;
int start()
{
int Cur_Hour=Hour();
double Cur_Min =Minute();
double Cur_time=Cur_Hour + Cur_Min100;
Alert(Cur_time);
if (Cur_time>=Time_Cls)
Executor();
return;
}
int Executor()
{
if (Flag_Time==false)
{
Alert("Important news time. Close orders.");
Flag_Time=true;
}
return;
}
The server time is calculated in hours and minutes during the execution of the special function start() (blocks 2-3). The line:
double Cur_time = Cur_Hour + Cur_Min100;
represents the current server time expressed as the real variable
Cur_time. The use of real variables is convenient in comparison
operations:
if (Cur_time >= Time_Cls)
If the current time is more than or equal to the value of Time_Cls
specified by user, then the Executor() user-defined function will be called
for execution. In this example, the user-defined function puts out a
message with trading recommendations. In general, this function may
contain any code, for example, make trades, send e-mails, create
graphical objects, etc.
Functions of Date and Time
Function |
Description
|
Day |
It returns the current day of month, i.e. the day of month of the last known server time.
|
DayOfWeek |
It returns the index number of the day of week (sunday-0,1,2,3,4,5,6) of the last known server time. |
DayOfYear |
It returns
the current day of year (1 is the 1st of January,..,365(6) is the 31st
of December), i.e. the day of year of the last known server time. |
Hour |
It returns
the current hour (0,1,2,..23) of the last known server time at the
moment of program start (the value is not changed during the execution
of the program).
|
Minute |
It returns
the current minute (0,1,2,..59) of the last known server time at the
moment of program start (the value is not changed during the execution
of the program).
|
Month |
It returns
the number of the current month (1-January,2,3,4,5,6,7,8,9,10,11,12),
i.e. the number of the month of the last known server time. |
Seconds |
It returns
the number of seconds lapsed since the beginning of the current minute
of the last known server time at the moment of program start (the value
is not changed during the execution of the program). |
TimeCurrent |
It returns the last known server time (the time of last quote coming) expressed in the number of seconds that passed
since the 00:00 January 1-st of 1970. |
TimeDay |
It returns the day of month (1 - 31) for the specified date. |
TimeDayOfWeek |
It returns the day of week (0-Sunday,1,2,3,4,5,6) for the specified date. |
TimeDayOfYear |
It returns the day (1 is the 1st of January,..,365(6) is the 31st of December) of year for the specified date. |
TimeHour |
It returns the hour for the specified time. |
TimeLocal |
It returns the local PC time expressed in the number of seconds lapsed since 00:00 of the 1st of January 1970. |
TimeMinute |
It returns minutes for the specified time. |
TimeMonth |
It returns the number of the month for the specified time (1-January,2,3,4,5,6,7,8,9,10,11,12).
|
TimeSeconds |
It returns the number of seconds passed from the beginnig of the specified time. |
TimeYear |
It returns the year for the specified date. The returned value can be within the range of 1970-2037. |
Year |
It returns the current year, i.e. the year of the last known server time. |
To get the detailed information about these and other functions, refer to the Documentation at MQL4.community, at MetaQuotes Ltd. website or at the "Help" section of MetaEditor.