Operator 'continue'
Sometimes, when you use a cycle in the code, it is necessary to early terminate processing of the
current iteration and go to the next one without executing the remaining operators that compose the cycle body. In such cases, you should use the operator 'continue'.
Format of the Operator 'continue'
The operator 'continue' consists of one word and ends in ";" (semicolon).
continue; // Operator 'continue'
Execution Rule of the Operator 'continue'
 |
The operator 'continue' stops the execution of the current iteration of the nearest cycle operator
'while' or 'for'. The execution of the operator 'continue' results in going to the next iteration of the nearest cycle operator 'while' or 'for'. The operator 'continue' can be used only in the body of the above cycle operators. |
Let's consider how the operator 'continue' can be practically used.
 |
Problem 16. There are 1000 sheep on the first farm. The amount of sheep on the first farm increases by 1% daily. If the amount of sheep exceeds 50 000 at the end of month, then 10% of sheep will be transferred to the second farm. Within what time will the amount of sheep on the second farm reach 35 000? (We consider that there are 30 working days in a month.) |
The algorithm of solving this problem is obvious: We should organize a cycle where the program would calculate the total amount of sheep on the first farm. According to the problem statement, the sheep are transferred to the second farm at the end of month. It means that we will have to create one more (internal) cycle where the accumulation of sheep in the current month would be calculated. Then we will have to check at the end of month, whether the limit of 50 000 sheep is exceeded or not. If yes, then we should calculate the amount of sheep to be transferred to the second farm at the end of month
and the total amount of sheep on the second farm.
The algorithm under consideration is realized in script
sheep.mq4. The operator 'continue' is used here to make calculations in the external cycle.
int start()
{
int
day,
Mons;
double
One_Farm =1000.0,
Perc_day =1,
One_Farm_max=50000.0,
Perc_exit =10,
Purpose =35000.0,
Two_Farm;
while(Two_Farm < Purpose)
{
for(day=1; day<=30; day++)
One_Farm=One_Farm*(1+Perc_day/100);
Mons++;
if (One_Farm < One_Farm_max)
continue;
Two_Farm=Two_Farm+One_Farm*Perc_exit/100;
One_Farm=One_Farm*(1-Perc_exit/100);
}
Alert("The aim will be attained within ",Mons," months.");
return;
}
At the start of the program, as usually, variables are described and commented. The calculation itself is performed in the cycle 'while'. After it has been executed, the corresponding message is displayed. The calculations in the external cycle 'while' will be executing, until the aim is attained, namely, until the total amount of sheep on the second farm reaches the expected value of 35 000.
The internal cycle 'for' is very simple: the value of the balance daily increases by 1%.
No sum analysis is performed in this cycle, since, according to the problem statement, sheep can only be transferred at the end of month. Thus, after exiting the cycle 'for', variable One_Farm has the value that is equal to the amount of sheep on the first farm. Immediately after that, the value of variable Mons is calculated, which increases by 1 at execution of each iteration of the external cycle 'while'.
According to the current amount of sheep on the first farm, one of two actions below shall be performed:
- if the amount of sheep on the first farm exceeds the limit value of 50 000, then 10% of sheep of the first farm should be transferred to the second farm;
- otherwise, the sheep from the first farm stay on the first farm and are bred further.
The algorithm is branched using the operator 'if':
if (One_Farm < One_Farm_max)
continue;
These lines of the code can be characterized as follows: If the amount of sheep on the first farm
is below the limiting value of 50 000, then execute the operator composing the body of the operator 'if'. At the first iteration, the amount of sheep on the first farm turns out to be less than the limiting value,
so the control will be passed to the body of the operator 'if', i.e., to the operator 'continue'.
The execution of the operator 'continue' means the following: Terminate the current iteration of the nearest executing cycle (in this case, it is the cycle in the operator 'while') and pass the control to the header of the cycle operator. The following program lines that are also a part of the cycle operator-'while' body will not be executed:
Two_Farm = Two_Farm+One_Farm*Perc_exit/100;
One_Farm = One_Farm*(1-Perc_exit/100);
In these above lines, the reached amount of sheep on the second farm and the remaining sheep on the first farm are calculated. It is impossible to make these calculations at the first iteration, since the limit of 50 000 sheep on the first farm has not been reached yet. It is the essence of the operator 'continue' that consists in skipping these lines without executing them in the current iteration.
The execution of the operator 'continue' results in passing the control to the header of the cycle operator 'while'. Then the condition in the cycle operator is tested and the next iteration starts. The cycle will continue to be executed according to the same algorithm,
until the amount of sheep on the first farm reaches the predefined limit. The values of Mons are accumulated at each iteration.
At a certain stage of calculating, the amount of sheep on the first farm will reach or exceed the predefined limit of 50 000 head. In this case, at the execution of the operator 'if', the condition (One_Farm < One_Farm_max) becomes false, so the control will not be passed to the body of the operator 'if'. This means that the operator 'continue' will not be executed, whereas the control will be passed to the first of the last two lines of the cycle-'while' body: First, the program will calculate the amount of sheep on the second farm and then the remaining amount of sheep on the first farm. After these calculations have been completed, the iteration of the cycle 'while' will be finished and the control will be passed to the cycle header again. Below is the block diagram of the algorithm realized in script
sheep.mq4.
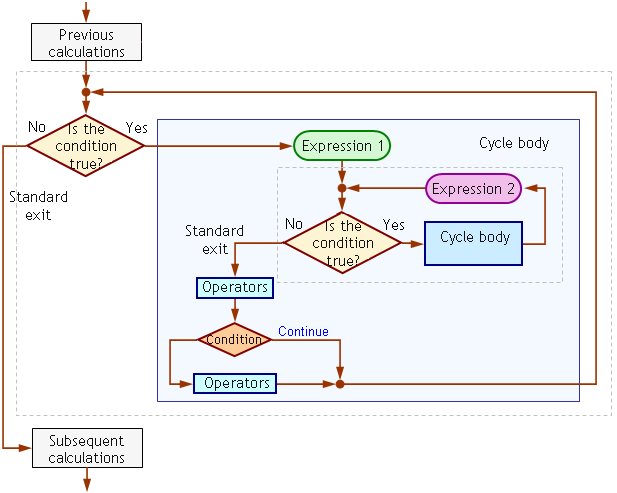
Fig. 47. Block diagram of a program where the operator 'continue' breaks iterations of the external cycle (sheep.mq4).
In the diagram, we can see that, depending on the execution of the condition in the operator 'if',
the control will be immediately passed to the header of the cycle 'while' (as a result of execution of the operator 'continue'), or some operators are executed first, and then the control will still be passed to the header of the cycle 'while'. In both cases, the execution of the cycle does not end.
 |
Please note that the presence of the operator 'continue' in the cycle body does not necessarily result in termination of the current iteration. This only happens if it executes, i.e., if the control is passed to it. |
At each next iteration in the cycle operator 'while', the new reached value of variable Two_Farm will be calculated. As soon as this value exceeds the predefined limit (35 000), the condition in the cycle operator 'while' will become false, so the calculations in the cycle-'while body will not be performed any longer, whereas the control will be passed to the nearest operator that follows the cycle operator:
Alert("The aim will be attained within ",Mons," months.");
The execution of the function Alert() will result in displaying of the following line:
The aim will be attained within 17 months. |
The operator 'return' completes execution of the special function start(), which results in that the control is passed to the client terminal, whereas the execution of the script is completed, it will be unloaded from the symbol window.
The algorithm above also provides a standard exit from the cycle 'while'. In a more general case, the exit from the cycle can result from the execution of the operator 'break' (special exit). However, neither one nor another way to close the cycle is related to interruption of the current iteration using the operator 'continue'.
In the execution rule of the operator 'continue' the phrase of "the nearest operator" is used. It does not mean that the program lines are close to each other.
Let's have a look at the code of script
sheep.mq4. The operator 'for' is "closer" to the operator 'continue' than the header of the cycle 'while'. However, this does not mean anything: The operator 'continue' has no relation to the cycle 'for', because it is outside its body. In general case, a program can contain multiple nested cycle operators. The operator 'continue' is always in the body of one of them. At the same time, the operator 'continue', as a part of the internal cycle operator, is, of course, also inside the external cycle operator. The phrase of "stops the execution of the current iteration of the nearest cycle operator"
should mean that the operator 'continue' influences the nearest cycle operator, within the body of which the operator 'continue' is located. In order to visualize it, let's slightly change the problem statement.
 |
Problem 17. There are 1000 sheep on one farm. The amount of sheep on this first farm increases daily by 1%. On the day, when the amount of sheep on the first farm reaches
50 000, 10% of sheep will be transferred to the second farm. Within what time will the amount of sheep on the second farm reach 35
000? (We consider that there are 30 working days in a month.)
|
The solution of problem 17 is realized in script
othersheep.mq4. In this case, the operator 'continue' is used for calculations in both the external and internal cycles.
int start()
{
int
day,
Mons;
double
One_Farm =1000.0,
Perc_day =1,
One_Farm_max=50000.0,
Perc_exit =10,
Purpose =35000.0,
Two_Farm;
while(Two_Farm < Purpose)
{
for(day=1; day<=30 && Two_Farm < Purpose; day++)
{
One_Farm=One_Farm*(1+Perc_day/100);
if (One_Farm < One_Farm_max)
continue;
Two_Farm=Two_Farm+One_Farm*Perc_exit/100;
One_Farm=One_Farm*(1-Perc_exit/100);
}
if (Two_Farm>=Purpose)
continue;
Mons++;
}
Alert("The aim will be attained within ",Mons," months and ",day," days.");
return;
}
To solve the problem, we have to analyze the amount of sheep for each day.
For this purpose, the lines:
if (One_Farm < One_Farm_max)
continue;
Two_Farm=Two_Farm+One_Farm*Perc_exit/100;
One_Farm=One_Farm*(1-Perc_exit/100);
are transferred into the internal cycle organized for the days of month. It is obvious that a certain amount of sheep are transferred to the second farm, in this case, not at the end of month, but on the day, when the amount of sheep on the first farm reaches the predefined value. The reason for using here the operator 'continue' is the same: We want to skip the lines containing the calculations related to the transfer of sheep from one farm to another, i.e., we don't want to execute these lines. Please note that the cycle 'while' constructed for the days of month is not interrupted independently on whether the sheep were transferred or not, because the operator 'continue' influences the nearest cycle operator, in our case, the cycle operator 'for'.
We used a complex expression as the Condition in the cycle operator 'for':
for(day=1; day<=30 && Two_Farm<Purpose; day++)
Using the operation && ("and") means that the cycle will be executed as long as both conditions are true:
- the month has not ended yet, i.e., the variable 'day' ranges from 1 through 30; and
- the amount of sheep on the second farm has not reached the predefined value yet, i.e., the variable Two_Farm
is less than Purpose (35 000).
If at least one of the conditions is not met (becomes false), the cycle stops.
If the execution of the cycle 'for' is stopped (for any reason), the control is passed to the operator 'if':
if (Two_Farm >= Purpose)
continue;
Mons++;
The use of the operator 'continue' at this location in the code has a simple sense: The program must continue counting months only if the amount of sheep on the second farm is below the expected value. If the aim has already been attained, the value of the variable Mons will not change,
whereas the control (as a result of the execution of 'continue') will be passed to the header of the nearest cycle operator, in our case, of the cycle operator 'while'.
The use of operators 'continue' in nested cycles is shown in Fig. 48 below.
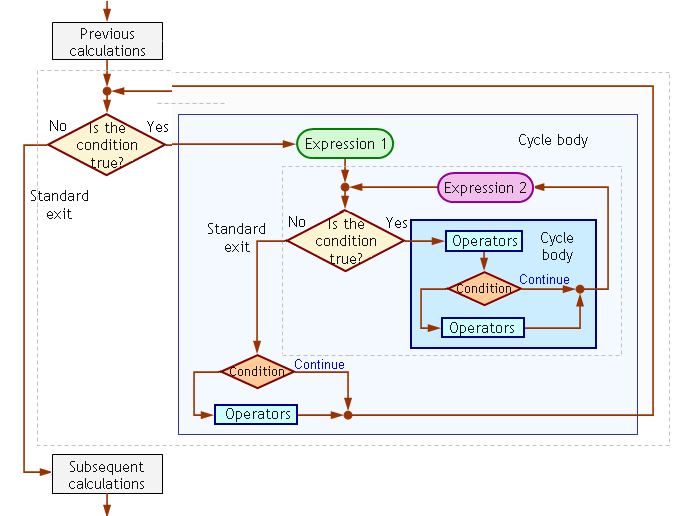
Fig. 48. Block diagram of the algorithm based on nested cycles. Each cycle body contains its operator 'continue' (othersheep.mq4).
In the above example, each cycle (external and internal) has one operator 'continue' that influences only its "own" nearest cycle,
but not any events in any other cycle. Generally, in a cycle body, several operators 'continue' can be used, each being able to interrupt the current iteration as a result of meeting of a condition. The amount of operators 'continue' in a cycle body is not limited.