Function Defining Trading Criteria
The success of any trading strategy mainly depends on the sequence of trading criteria calculations. The function that defines trading criteria is the most important part of a program and must be used without fail. According to trading strategy, the function may return values that correspond with particular trading criteria.
In a general case, the following criteria can be defined:
- criterion for opening of a market order;
- criterion for closing of a market order;
- criterion for partly closing of a market order;
- criterion for closing of opposite market orders;
- criterion for modification of the requested prices of stops of a market order;
- criterion for placing of a pending order;
- criterion for deletion of a pending order;
- criterion for modification of the requested open price of a pending order;
- criterion for modification of the requested prices of stops of a pending order.
In most cases, the triggering of one trading criterion is exclusive as related to other trading criteria. For example, if the criterion for opening a Buy order becomes important at a certain moment, this means that the criteria used for closing Buy orders or for opening Sell orders cannot be important at the same moment (see Relation of Trading Criteria). At the same time, according to the rules inherent in a given trading strategy, some criteria may trigger simultaneously. For example, the criteria for closing a market order Sell and for modification of a pending order BuyStop may become important simultaneously.
A trading strategy imposes requirements to the content and the usage technology of the function defining trading criteria. Any function can return only one value. So, if you have realized in your Expert Advisor a trading strategy that implies using only mutually exclusive trading criteria, the value returned by the function can be associated with one of the criteria. However, if your strategy allows triggering of several criteria at a time, their values must be passed to other functions for being processed, using global variables for that.
Trading strategy realized in the EA below implies using only mutually exclusive criteria. This is why the function Criterion()
for passing the above criteria to other functions uses the value returned by the function.
User-Defined Function Criterion()
int Criterion()
The function calculates trading criteria. It can return the following values:
10 - triggered a trading criterion for closing of market order Buy;
20 - triggered a trading criterion for opening of market order Sell;
11 - triggered a trading criterion for closing of market order Buy;
21 - triggered a trading criterion for opening of market order Sell;
0 - no important criteria available;
-1 - the symbol used is not EURUSD.
The function uses the values of the following external variables:
St_min - the lower level of indicator Stochastic Oscillator;
St_max - the upper level of indicator Stochastic Oscillator;
Open_Level - the level of indicator MACD (for order opening);
Close_Level - the level of indicator MACD (for order closing).
In order to display messages, the function uses the data function Inform().If the function Inform() is not included in the EA, no messages will be displayed.
Function defining trading criteria, Criterion(), is formed as include file Criterion.mqh:
extern int St_min=30;
extern int St_max=70;
extern double Open_Level =5;
extern double Close_Level=4;
int Criterion()
{
string Sym="EURUSD";
if (Sym!=Symbol())
{
Inform(16);
return(-1);
}
double
M_0, M_1,
S_0, S_1,
St_M_0, St_M_1,
St_S_0, St_S_1;
double Opn=Open_Level*Point;
double Cls=Close_Level*Point;
M_0=iMACD(Sym,PERIOD_H1,12,26,9,PRICE_CLOSE,MODE_MAIN,0);
M_1=iMACD(Sym,PERIOD_H1,12,26,9,PRICE_CLOSE,MODE_MAIN,1);
S_0=iMACD(Sym,PERIOD_H1,12,26,9,PRICE_CLOSE,MODE_SIGNAL,0);
S_1=iMACD(Sym,PERIOD_H1,12,26,9,PRICE_CLOSE,MODE_SIGNAL,1);
St_M_0=iStochastic(Sym,PERIOD_M15,5,3,3,MODE_SMA,0,MODE_MAIN, 0);
St_M_1=iStochastic(Sym,PERIOD_M15,5,3,3,MODE_SMA,0,MODE_MAIN, 1);
St_S_0=iStochastic(Sym,PERIOD_M15,5,3,3,MODE_SMA,0,MODE_SIGNAL,0);
St_S_1=iStochastic(Sym,PERIOD_M15,5,3,3,MODE_SMA,0,MODE_SIGNAL,1);
if(M_0>S_0 && -M_0>Opn && St_M_0>St_S_0 && St_S_0<St_min)
return(10);
if(M_0<S_0 && M_0>Opn && St_M_0<St_S_0 && St_S_0>St_max)
return(20);
if(M_0<S_0 && M_0>Cls && St_M_0<St_S_0 && St_S_0>St_max)
return(11);
if(M_0>S_0 && -M_0>Cls && St_M_0>St_S_0 && St_S_0>St_min)
return(21);
return(0);
}
In block 1-2, the values returned by the function are described. In block 2-3, some external variables are declared. The include file Criterion.mqh is the only file used in the considered EA, in which the global (in this case, external) variables are declared. In the section named Structure of a Normal Program, you can find the reasoning for declaring of all global variables without exception in a separate file Variables.mqh. In this case, the external variables are declared in the file Criterion.mqh for two reasons: first, to demonstrate that it is technically possible (it is not always possible); second, to show how to use external variables at debugging/ testing of a program.
It is technically possible to declare external variables in the file Criterion.mqh, because these variables are not used in any other functions of the program.
The values of external variables declared in block 2-3 determine the levels for indicators
Stochastic Oscillator and MACD and are used only in the considered function Criterion().
The declaration of external variables in the file containing the function that defines trading criteria
may be reasonable, if the file is used temporarily,
namely, during the program debugging and calculating of optimal values of those external variables. For this purpose, you can add other external variables in the program, for example, to optimize inputs of indicators (in this case, the constants set the values of 12,26,9 for MACD and 5,3,3 for Stochastic Oscillator). Once having finished coding, you may delete these external variables from the program and replace them with constants with the values calculated during optimization.
In block 3-4, the local variables are opened and described. The Expert Advisor is intended to be used
on symbol EURUSD, so the necessary check is made in block 3-4. If the EA is launched in the window of another symbol, the function finishes operating and returns the value of -1 (wrong symbol).
In the program, the values of two indicators calculated on the current and on the preceding bar are used (block 4-5). Usually, when you use indicators Stochastic Oscillator and MACD, the signals for buying or selling are formed when two indicator lines meet each other. In this case, we use two indicators simultaneously to define trading criteria. The probability of simultaneous intersection of indicator lines of two indicators is rather low. It is much more probable that they will intersect one by one - first in one indicator, a bit later - in another one. If the indicator lines intersect within a short period of time, two indicators can be considered to have formed a trading criterion.
For example, below is shown how a trading criterion for buying is calculated (block 5-6):
if(M_0>S_0 && -M_0>Opn && St_M_0>St_S_0 && St_S_0<St_min)
According to this record, the criterion for buying is important if the following conditions are met:
- in indicator MACD, indicator line MAIN (histogram) is above indicator line SIGNAL and below the lowest level Open_Level (Fig. 157);
- in indicator Stochastic Oscillator, indicator line MAIN (histogram) is above indicator line SIGNAL and below the lowest level St_min (Fig. 158).
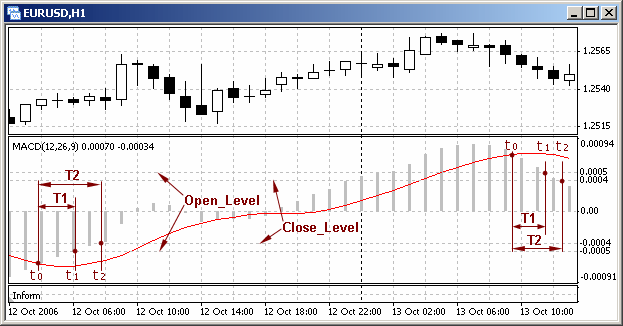
Рис. 157. Necessary condition of MACD indicator line positions to confirm the importance of trading criteria for opening and closing of orders.
In the left part of Fig. 157, the positions of MACD indicator lines is shown, at which two criteria trigger - opening of Buy and closing of Sell. Indicator line MAIN is below the level of 0.0005 within the time of T1 = t 1 - t 0. If the necessary indications of Stochastic Oscillator occur at this moment, the criterion for opening of Buy will trigger. Line MAIN is below the level of 0.0004 within the time T2 = t 2 - t 0. If the indications of Stochastic
Oscillator confirm this position, the criterion for closing of Sell will trigger.
Please note that, formally, both criteria above trigger within T1 (if confirmed by Stochastic
Oscillator). It was mentioned before that the considered function Criterion() returns only one value, namely, the value assigned to one triggered criterion. During this period, it becomes necessary to choose one of the criteria. This problem is solved in advance, during programming, according to the priorities prescribed by the trading strategy.
In this case (according to the trading strategy considered), the priority of opening a Buy order is higher than that of closing a Sell order. This is why, in block 5-6, the program line, in which the criterion for opening of Buy, is positioned above. If during the period of T1 (Fig. 157), we have got the confirmation from Stochastic
Oscillator, the function returns 10 assigned to this criterion. Within the period from t1 to t2, the function will return
21 assigned to the Sell closing criterion.
At the same time, at the execution of trade functions, the necessary trade requests will be formed. At triggering of the criterion for opening of Buy, first of all, the trade requests for closing of all available Sell orders will be formed. As soon as no such orders are left, opening of one Buy order will be requested. Respectively, when the criterion for closing of Sell orders triggers, a sequence of trade requests for only closing of all Sell orders will be formed (see Trade Functions).
The conditions, at which Stochastic Oscillator confirmation triggers,
are shown in Fig. 158.
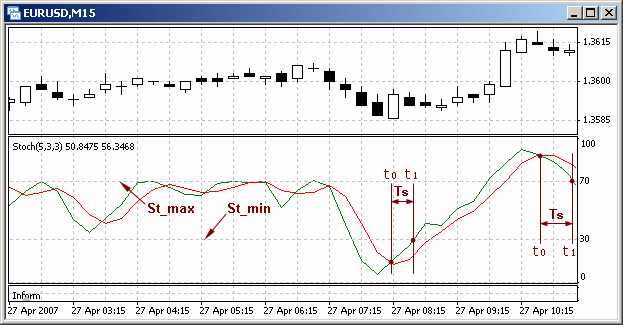
Fig. 158. Necessary condition of Stochastic Oscillator indicator line positions to confirm the importance of trading criteria for opening and closing of orders.
According to the program code specified in block 5-6, the criteria for opening of Buy and closing of Sell can become important provided the indicator line MAIN turns out to be above the signal line SIGNAL in Stochastic
Oscillator, line MAIN being below the minimum level St_min. In Fig. 158, such conditions are formed within the period of Ts. The mirrored conditions confirm the triggering of criteria for opening of the order Sell and closing of the order Buy (in the right part of Fig. 158). If no criterion has triggered, the function returns 0.
Other trades can be made under these conditions, for example, correction of the stop levels requested.
It must be noted separately that the considered trading strategy implies the usage of indications produced by MACD calculated on the one-hour timeframe, whereas Stochastic Oscillator
is calculated on the 15-minute timeframe.The timeframe may be changed during testing in order to optimize the strategy. However, after testing, in the final code of the function Criterion(), it is necessary to specify constant value for all parameters calculated,
including timeframes. The EA must be used only under the conditions, for which it has been created. In the example above (with the values of PERIOD_H1 and PERIOD_M15 specified explicitly in the indicators), the EA will consider only necessary parameters regardless the current timeframe set in the symbol window, where the EA has been launched.
 |
Trading criteria accepted in this given EA are used for training purposes and should not be considered as a guide to operations when trading on a real account. |